Lab 2: System Verilog
Purpose
This is a crash course in basic System Verilog constructs. As some level of exposure to Verilog is a requirement for access to the team, this lab consists of a series of exercises that will ensure you’ve got the basics on lock.
If you find there is a construct or challenge you’re unsure how to approach, seek guidance from the team. Asking questions is encouraged.
Setup
Fork the Lab Week 2 repo to your personal Github account and complete the exercises in that repo. (For design log purposes, this cloned repo will be the one you link to.)
The toolchain has already been setup for you for all the exercises. It uses techniques you have not been introduced to yet so don’t be concerned if you don’t understand all of it. You can build the simulations using the techniques from the Week 1 Onboarding Lab.
If you wish to test your work, you can do so by running the following command inside your build directory:
ctest --output-on-failure .
We’ll talk more about setting up and running tests in the next lab.
Exercise 1: Warm Up
Implement the following opcode table with a single combinational assign
statement.
op | out |
---|---|
0 | a + b |
1 | a - b |
2 | a & b |
3 | a | b |
Exercise 2: Sequential Logic
Implement an xor-based Fibonacci Linear Feedback Shift Register with taps at bits 15, 13, 12, and 10 (schematic provided below). If the reset is low, the LFSR should be initialized with the value present on the init input bus.
Step the output on positive clock edge.
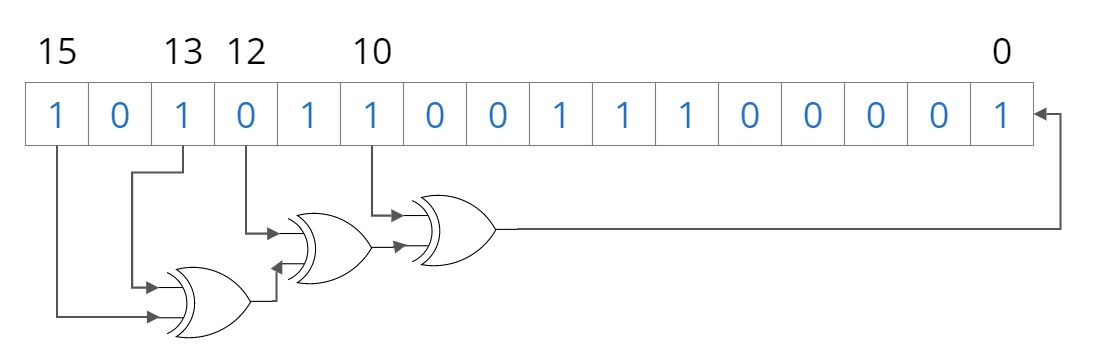
Exercise 3: Module Composition
There are two Mystery modules under the Mysteries directory. Compose them according to the following schematic:
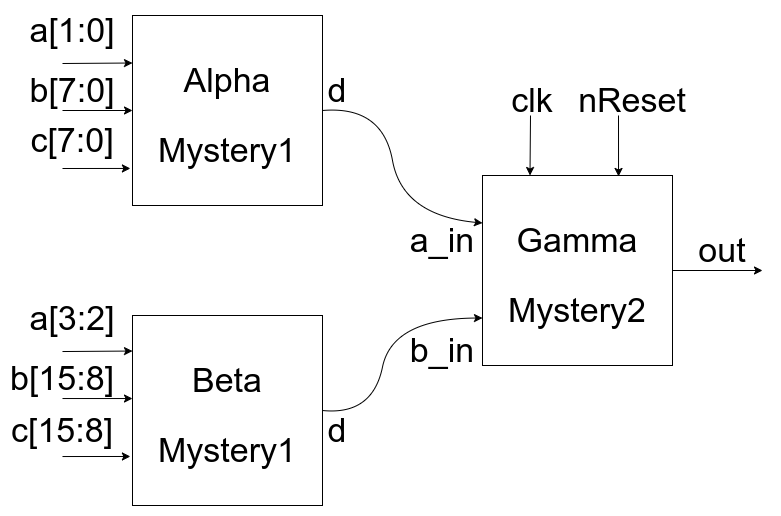
Make Note: In the next lab you’ll learn more about verification testing, but
for now take a quick glance at dv/exercise1.cpp
, dv/exercise2.cpp
, and
dv/exercise3.cpp
. Exercise1 exhaustively tests all possible inputs,
Exercise2 samples some possible initial values for a hundred cycles, and
Exercise3 randomly tests inputs for a hundred cycles.
Why do you think the reason for these different testing approaches are? What are their advantages and disadvantages?
Exercise 4: Combinational Logic
Using an always_comb
block, implement a decoder/mux that implements the
behavior described in the following table.
cs | sel | out |
---|---|---|
0 | X | 0 |
1 | 0 | alpha |
1 | 1 | beta |
1 | 2 | gamma |
1 | 3 | 0 |